In this article I'm going to give a step-by-step solution to Google Chromebook logic puzzle describing my exact thought process and hopefully providing few useful pieces of advice regarding cracking puzzles in general. However I encourage you to have a go at the puzzle yourself before reading the solution.
EDIT: Unfortunately Google took the challenge offline so you can't play it anymore. Meh.
The Begining
We begin with guesstimating the correct order for colourful balls. This is the easy part, it's pretty much a Mastermind game, but I didn't realize it at that time. There is no limit on the number of attempts and you can pretty much brute force it. Each time you attempt to make a guess, you are being given a 'hint' or a 'score' on how close was your attempt to the actual solution. After trying for couple of times you can deduct what's the meaning of these little black/white/gray bulbs even if you've never seen a Mastermind game before.
Here is how I started the game:
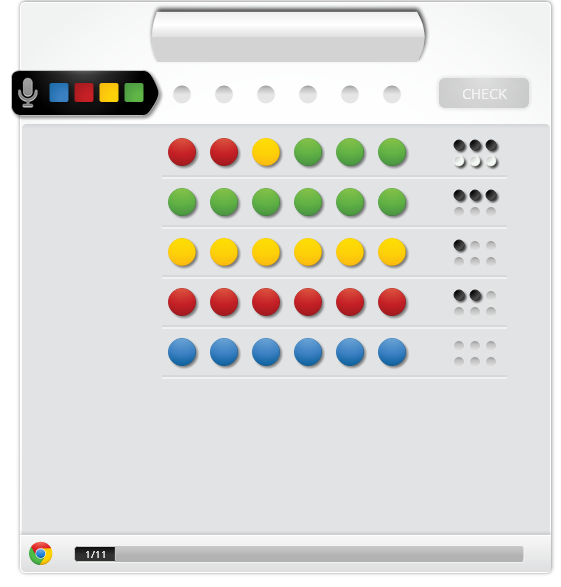
Terminology: What in Mastermind is called code pegs I ignorantly refer to as 'balls' within this document. What in Mastermind would be key pegs I simply call 'bulbs'.
After each guess I'm getting a feedback consisting of 6 bulbs:
- each black bulb represents a single ball in correct spot
- each white bulb represents a single ball in incorrect spot i.e. a ball of this color should be used somewhere else in the sequence
- gray/empty bulb represents ball that has no place in the final answer
With no more than 4 attempts you can estimate how many times a ball of each color should appear in the final solution. That simplifies the problem to arranging the balls in correct order. I admit I pretty much brute forced the actual correct order and it took me roughly 20 minutes to go through all 11 levels while having the lunch in the office at the same time.
The Mysterious Code
After each level you're presented with a little hint, see the arrangements of bulbs below:
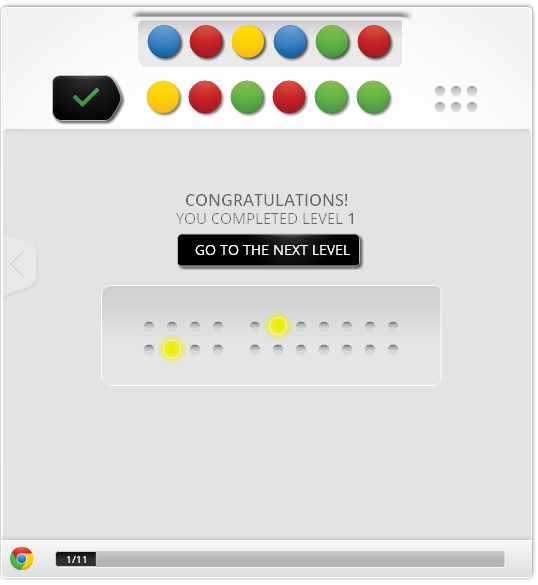
Not much huh? Well that's all the help you will get! After each level a screen similar to the one above is displayed. Eventually you will be asked if you've 'cracked the code' and to submit a solution. After finishing all 11 levels I've stopped for the moment an summarized my knowledge so far:
- There are 11 levels in total and 22 bulbs in 2 rows, 11 bulbs each
- Each level is associated with 2 lightened bulbs
- Lightened bulbs are unique for each level
- Each level is associated with 6 balls in one out of 4 colors
From the points listed above I've deducted the following:
- Bulbs represent some kind of 'meta data' - position, location, placement.
- The actual value must be represented by the colorful balls
- There are 6 balls and 2 highlighted bulbs per each level thus each 3 balls must represent a single 'unit' of data
Based on these assumptions I've tried to come up with some way (an algorithm) to derive a value from the set of 3 balls, usually by mixing and re-calculating their RGB representations in different ways. That didn't work.
So let's have another look at the screen above, there is one more hint on that screen which I've managed to completely ignore. On the very top of the screen there are 6 balls that are persistent between levels. It's easy to miss them because colors are quite familiar - it's Google logo colors. Let's make another assumption:
- The key to the solution is Google logo or its colors.
At this point my imagination went quite wild. I've tried to perform various calculations on RGB representations of Google logo bulbs and each level's solution bulbs. I've went as far as counting the number of occurrences of each color (blue and red -> 2, green and yellow ->1), converting that to binary and trying to get the answer that way. I've gave up after about an hour, finished lunch and get back to office work.
The Revelation
After a busy day in the office I've been chilling at home with some cold beer when a revelation struck me - I already had the algorithm I've been looking for so badly! The algorithm has been used to give me feedback after each guess in the initial Mastermind-like game. Let's try to recreate it:
- Iteratively compare a ball from the guessed answer to a bulb in the same spot in the secret solution
- If bulbs have the same colour, the result is black dot [•]
- If bulbs have different colour, check other bulbs in the secret solution. If there is one with a matching colour in the secret solution (that is not matched correctly in the guessed answer and it has not been used before) the result is white dot [◦], otherwise it's null [0].
- In case where operation 3 results in [◦], mark that bulb in secret solution as 'used'.
- After comparing all balls Count [•], [◦] and [0] and use sorted counts to give feedback
In practice this is how it would look like:
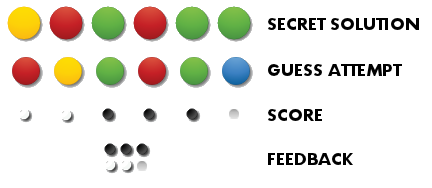
Let's re-use the same algorithm to score the level's 1 solution against Google colors:
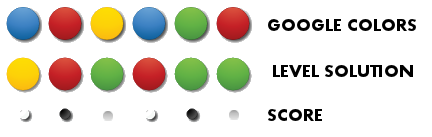
Let's reassess the assumptions made earlier on:
- Bulbs represent some kind of 'meta data' - position, location, placement.
- The actual value must be represented by the bulbs.
- There are 6 balls and 2 highlighted bulbs per each level thus each 3 balls must represent a single 'unit' of data
- The key to the solution is Google logo or its colors.
Based on assumptions 2 & 4 we've derived a value, marked as score on the chart above. Based on assumption 3 we need to derive 2 'units' of data. Earlier on feedback has been given in 2 rows, 3 bulbs each, so let's do the same - but without sorting (effectively skipping step 5 in the algorithm described above). We will have:
- [◦] [•] [0]
- [◦] [•] [0]
Same? Funny.
Now follows the tricky part - so far we've been speaking about balls and bulbs, however the final answer must be a number or sentence. I've asked myself a question - how can I convert these symbols into values? Well there are 3 possible values for each scoring operation ([•], [◦] or [0]). Let's assign these symbols numerical values - 2 for [•], 1 for [◦] and 0 for [0]. So far we have
- 1,2,0
- 1,2,0
120 in ascii is lowercase 'x', however if you think about it, most of the combinations of 0, 1 and 2 don't produce meaningful values in ascii (e.g. 012 would be 'vertical tab'). You can't build a sentence with such a limited vocabulary either. It's an incorrect approach, let's try something different.
With my scoring algorithm I can produce no more than 3 symbols, later converted into numeric values. Since I can have only 3 different values I shouldn't assume a decimal system! A Ternary (base 3) system would be more suitable, let's try it:
- 1203 = 1510
- 1203 = 1510
Ok... that still doesn't mean much in ascii. Let's see what kind of values I can expect with 3 digits ternary system:
- Minimum: 0003 = 010
- Maximum: 2223 = 2610
26? Hold on, isn't that a total number of letters in english alphabet? Wikipedia!
The modern English alphabet is a Latin-based alphabet consisting of 26 letters
Bingo! Lazy as I'm I've googled for english letters ordering numbers (got this) and looked up 15th letter of the alphabet (it's letter O). So we have:
- [◦] [•] [0] => 1,2,0 => 1203 = 1510 => 'O'
- [◦] [•] [0] => 1,2,0 => 1203 = 1510 => 'O'
Assumption no 1 says "Bulbs represent some kind of 'meta data' - position, location, placement". Let's try that:
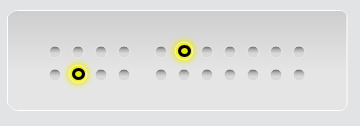
From that point it's couple of minutes to work your way through the remaining 10 levels reusing the same approach. Couple of minutes later I had the code cracked and submitted to Google. And hey! I've won a Chromebook :)
The Summary
Cracking logic puzzles like this one is not something you can learn. Yet having a correct approach can definitely help. Couple of hints I can share:
- Whenever you're stuck - stop and assess your knowledge. Write it down as a list, graph or something.
- If you're still stuck make an assumption and chase it. Gazing at the problem usually doesn't help.
- It's ok to fail. Learn from it, then discard a non-working solution, revert back to a known state and start again.
- If you're stuck for a long time, leave it. Look for something else to do and come back to a problem later on.
- Don't give up!